1. μμΈ μ²λ¦¬κ° 무μμΈκ°.
μμΈ (exception) : μλͺ»λ μ½λ , λΆμ νν λ°μ΄ν° , μμΈμ μΈ μν©μ μνμ¬ λ°μνλ μ€λ₯ (μ) 0μΌλ‘ λλλ κ²κ³Ό κ°μ μλͺ»λ μ°μ°μ΄λ λ°°μ΄μ μΈλ±μ€κ° νκ³λ₯Ό λμ μ λ μκ³ , λμ€ν¬μμλ νλμ¨μ΄ μλ¬κ° λ°
2. μμΈμ²λ¦¬ λ°©λ²
- μμΈλΆλΆμ νλνλ 쑰건 μ²λ¦¬ν¨

3. μμΈμ²λ¦¬ νμ
try-catch λΈλ‘
try : μμΈκ° λ°μν μ μλ μνν μ½λ
catch : μμΈλ₯Ό μ²λ¦¬νλ μ½λ
throw: μμΈ μν©μ΄ λ°μνλ Έμμ μ릴 λ μ¬μ©ν¨
//μμΈμ²λ¦¬κΈ° λ§€κ°λ³μ λͺ¨λ νμ
κ°λ₯ν¨
throw persons;
catch (...)
{
}
catch(TooSmallException e) {
//TooSmallExceptionλ§ μ‘νλ€.
}
//μμΈμ²λ¦¬κΈ° λ§€κ°λ³μ νμ
μΌμΉν΄μΌλ¨ !!
throw persons;
catch (int e)
{
}
catch(...) {
//TooSmallExceptionμ μ μΈν λλ¨Έμ§ μμΈλ€μ΄ μ‘νλ€.
}
int main()
{
int pizza_slices = 0;
int persons = -1;
int slices_per_person = 0;
try
{
cout << "νΌμ μ‘°κ°μλ₯Ό μ
λ ₯νμμ€: ";
cin >> pizza_slices;
cout << "μ¬λμλ₯Ό μ
λ ₯νμμ€: ";
cin >> persons; //μμΈμ²λ¦¬κΈ° λ§€κ°λ³μ νμ
μΌμΉν΄μΌλ¨
if (persons == 0)
throw persons;
slices_per_person = pizza_slices / persons;
cout << "νμ¬λλΉ νΌμλ " << slices_per_person << "μ
λ
λ€." << endl;
}
catch (int e) //μμΈμ²λ¦¬κΈ° λ§€κ°λ³μ νμ
μΌμΉν΄μΌλ¨
{
cout << "!!μ¬λμ΄ " << e << " λͺ
μ
λλ€. " << endl;
}
return 0;
}
//μμΈμ²λ¦¬κΈ° ν¨μλ‘ λ§€κ°λ³μλ₯Ό λ°λ
#include <iostream>
using namespace std;
int dividePizza(int pizza_slices, int persons);
int main()
{
int pizza_slices = 0;
int persons = 0;
int slices_per_person = 0;
try
{
cout << "νΌμ μ‘°κ°μλ₯Ό μ
λ ₯νμμ€: ";
cin >> pizza_slices;
cout << "μ¬λμλ₯Ό μ
λ ₯νμμ€: ";
cin >> persons;
slices_per_person = dividePizza(pizza_slices, persons);
cout << "νμ¬λλΉ νΌμλ " << slices_per_person << "μ
λ
λ€." << endl;
}
int dividePizza(int pizza_slices, int persons)
{
if (persons == 0)
throw persons;
return pizza_slices / persons ;}
catch (int e)
{
cout << "!! μ¬λμ΄ " << e << " λͺ
μ
λλ€. " << endl;
}
return 0;
}
//λ€μ€ catch λ¬Έ
try {
cout << "νΌμ μ‘°κ°μλ₯Ό μ
λ ₯νμμ€: ";
cin >> pizza_slices;
cout << "μ¬λμλ₯Ό μ
λ ₯νμμ€: ";
cin >> persons;
if (persons < 0) throw "negative"; // μμΈ λ°μ!
if (persons == 0) throw persons; // μμΈ λ°μ!
slices_per_person = pizza_slices / persons;
cout << "νμ¬λλΉ νΌμλ " << slices_per_person << "μ
λλ€." << endl;
}
catch (const char *e) {
cout << "μ€λ₯: μ¬λμκ° " << e << "μ
λλ€" << endl;
}
catch (int e) {
cout << "μ€λ₯: μ¬λμ΄ " << e << " λͺ
μ
λλ€." << endl;
}
4. ν νλ¦Ώ, ν΄λμ€ν νλ¦Ώ (λ§λ€λΌκ³ λμ¬μλμμ)
//ν¨μ ν
νλ¦Ώ
template <typename νμ
μ΄λ¦>
ν¨μμ μν
{
....// ν¨μμ 본체
}
//ν΄λμ€ ν
νλ¦Ώ
template <typenmae T>
class ν΄λμ€μ΄λ¦
{
...// Tλ₯Ό μ΄λμλ μ¬μ© κ°λ₯
}
template <typename T>
T get_sum(T x)
{
x = x + 10;
return x;
}
template <typename T>
T get_sum(T x, T y)
{
if (x > y) return x;
else return y;
}
int main()
{
cout << get_sum(3) << endl;
cout << get_sum(1.2, 3.9) << endl;
return 0;
}
#include <iostream>
using namespace std;
template <typename T>
class Box {
T data; // Tλ νμ
(type)μ λνλΈλ€.
public:
Box() { }
void set(T value) {
data = value;
}
T get() {
return data;
}
------------------------------------
int main()
{
Box<int> box; // ꡬ체ν
box.set(100);
cout << box.get() << endl; //100
Box<double> box1;
box1.set(3.141592);
cout << box1.get() << endl; //3.14~
return 0;
}
5. STL
1. μκ³ λ¦¬μ¦
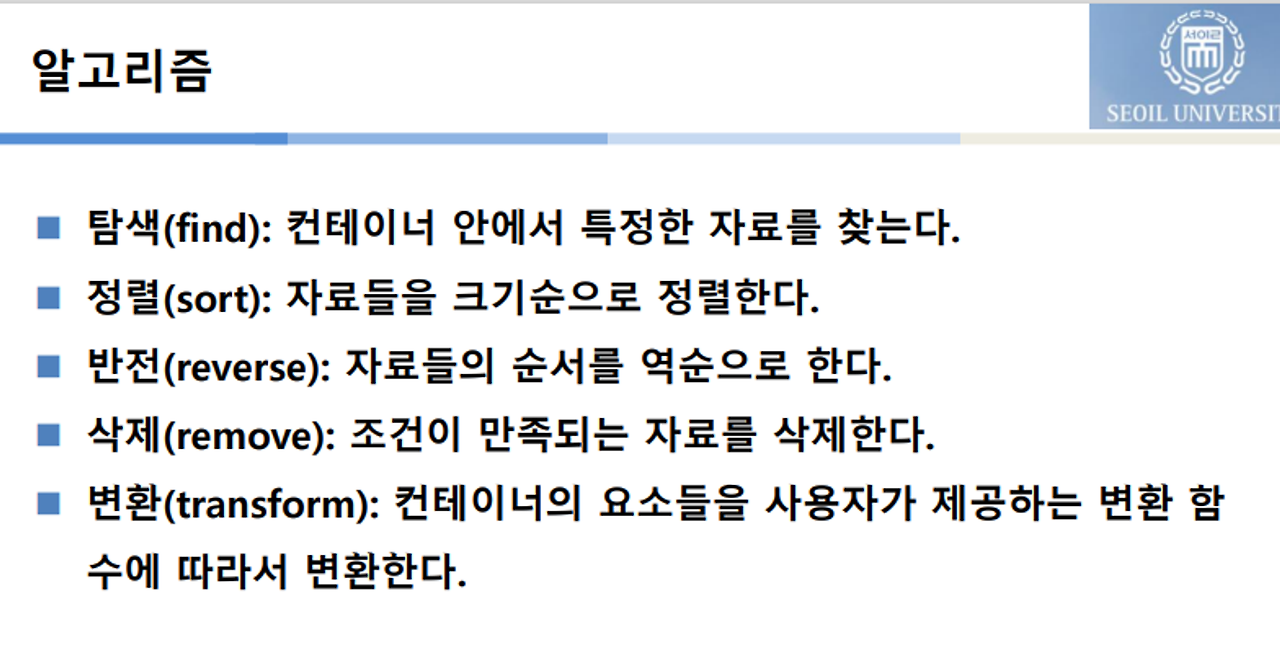
find() μ find_if()
count()
#include<algorithm>
int main()
{
vector<string> vec { "μ¬κ³Ό", "ν λ§ν ", "λ°°", "μλ°", "ν€μ" };
auto it = find(vec.begin(), vec.end(), "μλ°");
if (it != vec.end())
cout << "μλ°μ΄ " << distance(vec.begin(), it) << "μ μ
μ΅λλ€." << endl;
return 0;
}
#include<algorithm>
template <typename T>
bool is_even(const T& num)
{
return (num % 2) == 0;
}
int main()
{
vector<int> vec;
for (int i = 0; i<10; i++)
vec.push_back(i);
size_t n = count_if(vec.begin(), vec.end(), is_even<int>);
cout << "κ°μ΄ μ§μμΈ μμμ κ°μ: " << n << endl;
return 0;
binary_search()
for_each()
bool comp(string s1, string s2) {
return (s1 == s2);
}
int main(void) {
vector<string> v = { "one", "two", "three" };
bool result;
result = binary_search(v.begin(), v.end(), "two", comp);
if (result == true)
cout << "λ¬Έμμ΄ \"two\" μ λ²‘ν° μμ μμ." << endl;
return 0;
}
void printEven(int n) {
if (n % 2 == 0)
cout << n << ' ';
}
int main(void) {
vector<int> v = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
for_each(v.begin(), v.end(), printEven);
cout << endl;
return 0;
}
2.컨ν μ΄λ μλ£λ₯Ό μ μ₯νλ μ°½κ³ μ’ λ₯μ λ°λΌ μ μΆλ ₯ λ°©λ²μ΄ λ¬λΌμ§λ€.
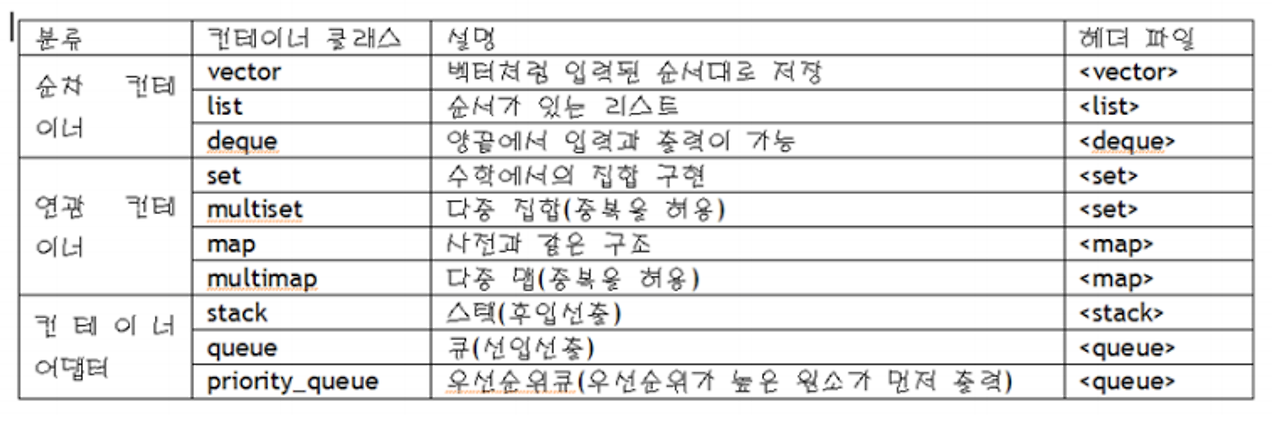
1.μμ°¨ 컨ν μ΄λ = μνμ€: βͺ μλ£λ₯Ό μμ°¨μ μΌλ‘ μ μ₯
⼠벑ν°(vector): λμ λ°°μ΄μ²λΌ λμνλ€. λ€μμ μλ£λ€μ΄ μΆκ°λλ€. (λμ λ°°μ΄ , μ€λ§νΈλ°°μ΄)
⼠리μ€νΈ(list): 벑ν°μ μ μ¬νμ§λ§ μ€κ°μμ μλ£λ₯Ό μΆκ°νλ μ°μ°μ΄ν¨μ¨μ μ΄λ€.
//리μ€νΈ
#include <list>
using namespace std;
int main()
{
list<int> my_list={ 10, 20, 30, 40 };
auto it = my_list.begin();
it++;
it++;
my_list.insert(it, 25);
for (auto& n : my_list)
cout << n << " ";
cout << endl
return 0;
}
βΌλ°ν¬(dequ): 벑ν°μ μ μ¬νμ§λ§ μμμλ μλ£λ€μ΄ μΆκ°λ μ μλ€
//λ± λ¬Έμμ΄ μ°μ°
#include <iostream>
#include <deque>
using namespace std;
int main()
{
deque<int> dq = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
dq.pop_front(); // μμμ μμ
dq.push_back(11); // λμμ μΆκ°
for (auto& n : dq)
cout << n << " ";
cout << endl;
return 0;
}
//λ± μ°μ°
#include <iostream>
#include <deque>
using namespace std;
int main()
{
deque<int> dq = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
dq.pop_front(); // μμμ μμ
dq.push_back(11); // λμμ μΆκ°
for (auto& n : dq)
cout << n << " ";
cout << endl;
return 0;
}
2. μ°κ΄ 컨ν μ΄λ = μ°κ΄μνμ€: βͺ μ¬μ κ³Ό κ°μ ꡬ쑰λ₯Ό μ¬μ©νμ¬μ μλ£λ₯Ό μ μ₯ βͺ μμλ€μ κ²μνκΈ° μν ν€(key) βͺ μλ£λ€μ μ λ ¬
βΌ μ§ν©(set): μ€λ³΅μ΄ μλ μλ£λ€μ΄ μ λ ¬λμ΄μ μ μ₯λλ€.
#include <set>
int main()
{
set<int> my_set;
my_set.insert(1);
my_set.insert(2);
my_set.insert(3);
auto pos = my_set.find(2);
if (pos != my_set.end())
cout << "κ° " << *pos << "κ° λ°κ²¬λμμ" << endl;
else
cout << "κ°μ΄ λ°κ²¬λμ§ μμμ" << endl;
return 0 ;
}
βΌ λ§΅(map): ν€-κ°(key-value)μ νμμΌλ‘ μ μ₯λλ€. ν€κ° μ μλλ©΄ ν΄λΉλλ κ°μ μ°Ύμ μ μλ€
ν€μ κ°μΌλ‘ μ΄λ£¨μ΄μ Έμμ (κ°κ°μ λμ λ리μ)
μμ΄μ¬μ ꡬνν¨, λ¨μ΄λ₯Ό λ°μμ λ¨μ΄μ μ€λͺ μ μΆλ ₯ν¨
#include <iostream>
#include <string>
#include <map>
using namespace std;
int main()
{
string word;
map<string, string> dic;
dic["boy"] = "μλ
";
dic["school"] = "νκ΅";
dic["office"] = "μ§μ₯";
dic["house"] = "μ§";
dic["morning"] = "μμΉ¨";
dic["evening"] = "μ λ
";
while (true) {
cout << "λ¨μ΄λ₯Ό μ
λ ₯νμμ€: ";
cin >> word;
if (word == "quit") break;
string meaning = dic[word];
if (meaning != "")
cout << word << "μ μλ―Έλ " << meaning << endl;
}
return 0;
}
#include <iostream>
#include <map>
#include <string>
#include <iterator>
using namespace std;
int main()
{
map<string, string> myMap;
myMap.insert(make_pair("κΉμ² μ", "010-123-5678"));
myMap.insert(make_pair("νκΈΈλ", "010-123-5679"));
myMap["μ΅μμ"] = "010-123-5680"
// λͺ¨λ μμ μΆλ ₯
for(auto& it : myMap){
cout << it.first << " :: " << it.second << endl;
}
if (myMap.find("κΉμν¬") == myMap.end())
cout << "λ¨μ΄ 'κΉμν¬'λ λ°κ²¬λμ§ μμμ΅λλ€. " << endl;
return 0;
}
3. 컨ν μ΄λ μ΄λν° βͺ μμ°¨ 컨ν μ΄λμ μ μ½μ κ°ν΄μ λ°μ΄ν°λ€μ΄ μ ν΄μ§ λ°©μμΌλ‘λ§ μ μΆλ ₯
βΌ μ€ν(stack): λ¨Όμ μ λ ₯λ λ°μ΄ν°κ° λμ€μ μΆλ ₯λλ μλ£ κ΅¬μ‘° (μ μ νμΆ)
#include <stack>
int main()
{
stack<string> st;
string sayings[3] =
{ "The grass is greener on the other side of the fence",
"Even the greatest make mistakes",
"To see is to believe" };
for (auto& s : sayings)
st.push(s);
while (!st.empty()) {
cout << st.top() << endl;
st.pop();
}
return 0;
}
βΌ ν(queue): λ°μ΄ν°κ° μ λ ₯λ μμλλ‘ μΆλ ₯λλ μλ£ κ΅¬μ‘° (μ μ μ μΆ) βΌ μ°μ μμν(priority queue): νμ μΌμ’ μΌλ‘ νμ μμλ€μ΄ μ°μ μ μλ₯Ό κ°μ§κ³ μκ³ μ°μ μμκ° λμ μμκ° λ¨Όμ μΆλ ₯λλ μλ£ κ΅¬μ‘°
include<queue>
int main()
{
queue<int> qu;
qu.push(100);
qu.push(200);
qu.push(300);
while (!qu.empty()) {
cout << qu.front() << endl;
qu.pop();
}
return 0;
}
3.λ°λ³΅μ
μΌλ°νλ ν¬μΈν°(generalized pointer) / μκ³ λ¦¬μ¦μ λ°λ³΅μλ₯Ό ν΅νμ¬ μ»¨ν μ΄λμ μ κ·Όνμ¬ μμ μ νλ€.
μμλ₯Ό μμ°¨μ μΌλ‘ μ²λ¦¬νκΈ°μν μ»΄ν¬λνΈ (for λ¬Έμμμ i λ λ°λ³΅μμ
- Begin() : 맨 μ²μλΆν°
- end() : 맨 λμμλΆν°
- ++ 컨ν μ΄λ λ€μμμ
- - - μ΄μ μμ
- = , ! = κ°μ μμλ₯Ό κ°λ¦¬ν€κ³ μλμ§ νμΈ
- * λ°λ³΅μκ° κ°λ¦¬ν€λ μμκ°μ μΆμΆνκΈ° μν μμ°Έμ‘° μ°μ°μ
//νμλ€μ μ±μ μ μ μ₯νλ 벑ν°λ₯Ό μμ±ν΄μ
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<double> scores (10);
for (int i=0; i<scores.size(); i++)
{
cout<<"μ±μ μ μ
λ ₯νμμ€:";
cin >> scores[i];
}
double highest =scores[0];
for(int i = 1; i<scores.size(); i++)
if (scores[i] > highest)
highest = scores[i];
cout<<"μ΅κ³ μ±μ μ" << highest << "μ
λλ€ \n";
return 0;
}
#include <iostream>
#include<vector>
using namespace std;
int main()
{
vector<double> scores;
while (true) {
double value = 0.0;
cout << "μ±μ μ μ
λ ₯ν΄ μ£ΌμΈμ :(-1μ μΉλ©΄ μ’
λ£) ";
cin >> value;
if (value < 0.0) break;
scores.push_back(value);
}
double highest = scores[0];
vector<double>::iterator it;
for(it = scores.begin(); it < scores.end(); it++)
if (*it > highest)
highest = *it;
cout << "μ΅κ³ μ μ±μ μ" << highest << "μ
λλ€." << endl;
return 0;
}
//5λ³΄λ€ ν° μ μ
bool is_greater_than_5(int value)
{
return (value > 5);
}
int main()
{
vector<int> numbers{ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
auto count = count_if(numbers.begin(), numbers.end(),is_greater_than_5);
cout << "5λ³΄λ€ ν° μ μλ€μ κ°μ: " << count << endl;
return 0;
}
6. λλ€μ & auto ν¨μ

//λλ€μ
#include <iostream>
using namespace std;
int main()
{
auto sum = [](int x, int y) { return x + y; };
cout << sum(1, 2) << endl;
cout << sum(10, 20) << endl;
return 0;
}
7. λ€νμ±(polymorphism)μ΄λ
8. μν₯/νν₯ νλ³ν
#include<iostream>
#include<list>
using namespace std;
class RemoteControl {
public:
virtual void turnOn() = 0;
virtual void turnOff() = 0;
};
class Television : public RemoteControl {
public:
void turnOn() {
cout << "tv on" << endl;
}
void turnOff() {
cout << "tv off" << endl;
}
void volCh() {
cout << "vol ch" << endl;
}
};
class Radio : public RemoteControl {
public:
void turnOn() {
cout << "tv on" << endl;
}
void turnOff() {
cout << "tv off" << endl;
}
void volAMFM() {
cout << "AMFM ch" << endl;
}
};
void main() {
RemoteControl* r1 = new Television();
r1->turnOn();
RemoteControl* r2 = new Radio();
r2->turnOn();
//r1->volCh(); λΆλͺ¨μ μλ κ²μ νν₯νλ³νμν΄μ€μΌλ¨ γ
γ
λΉμ°ν¨
((Television*)r1)->volCh();
/*void volCh() {
cout<<"RemoteControl vol ch"<<endl;
} μλ λΆλͺ¨ ν΄λμ€μ μΆκ° γ± */
return;
}
class Shape {
protected:
int x, y;
public:
void setOrigin(int x, int y){
this->x = x;
this->y = y;
}
void draw() {
cout <<"Shape Draw";
}
};
class Rectangle : public Shape {
private:
int width, height;
public:
void setWidth(int w) {
width = w;
}
void setHeight(int h) {
height = h;
}
void draw() {
cout << "Rectangle Draw";
}
}
class Circle : public Shape {
private:
int radius;
public:
void setRadius(int r) {
radius = r;
}
void draw() {
cout << "Circle Draw"<< endl;
}
};
void main() {
//Shape* pa = new Shape();//λ³Έλ λμ κ°μ²΄μμ±
//pa->draw();
Shape* pb = new Rectangle();//μν₯νλ³ν λΆλͺ¨
pb->draw();
((Rectangle*)pb) -> draw () ;// νν₯νλ³ν μμ
delete pb;
return;
}
class Shape {
...
}
class Rectangle : public Shape {
...
}
int main()
{
Shape *ps = new Rectangle(); // μν₯ νλ³ν
//μ¬κΈ°μ psλ₯Ό ν΅νμ¬ Rectangleμ λ©€λ²μ μ κ·Όνλ €λ©΄
ps->setOrigin(10, 10);
ps->draw(); //Shape ν¬μΈν°μ΄κΈ° λλ¬Έμ Shapeμ draw()κ° νΈμΆ
1. Rectangle *pr = (Rectangle *) ps;
2.((Rectangle *)ps)-> draw(); // νν₯ νλ³ν
delete ps;
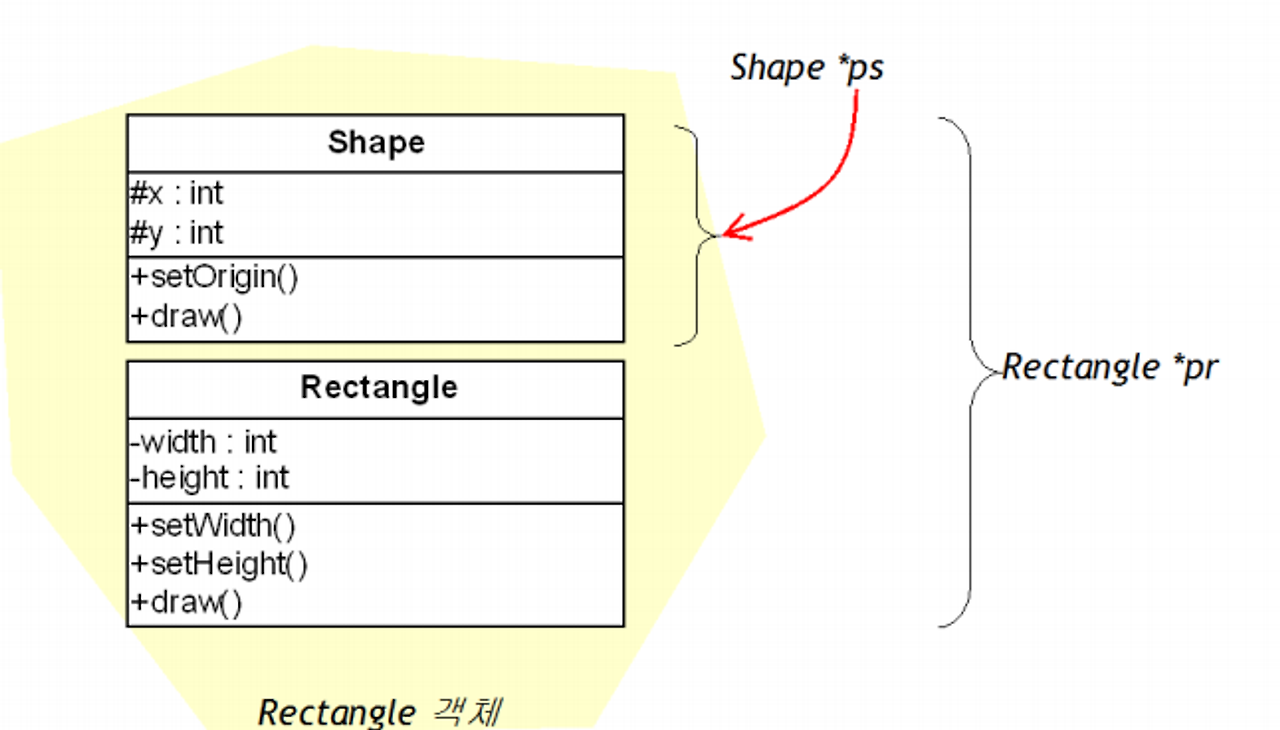
UML +
ν΄λμ€λͺ / μμ± (νΉμ§,λ³μ) / μ°μ°(λ©μλ)
-private +public #protected
λΆμλ¨κ³μ UML κ³Ό μ€κ³ λ¨κ³μ UMLμ΄ λ€λ¦
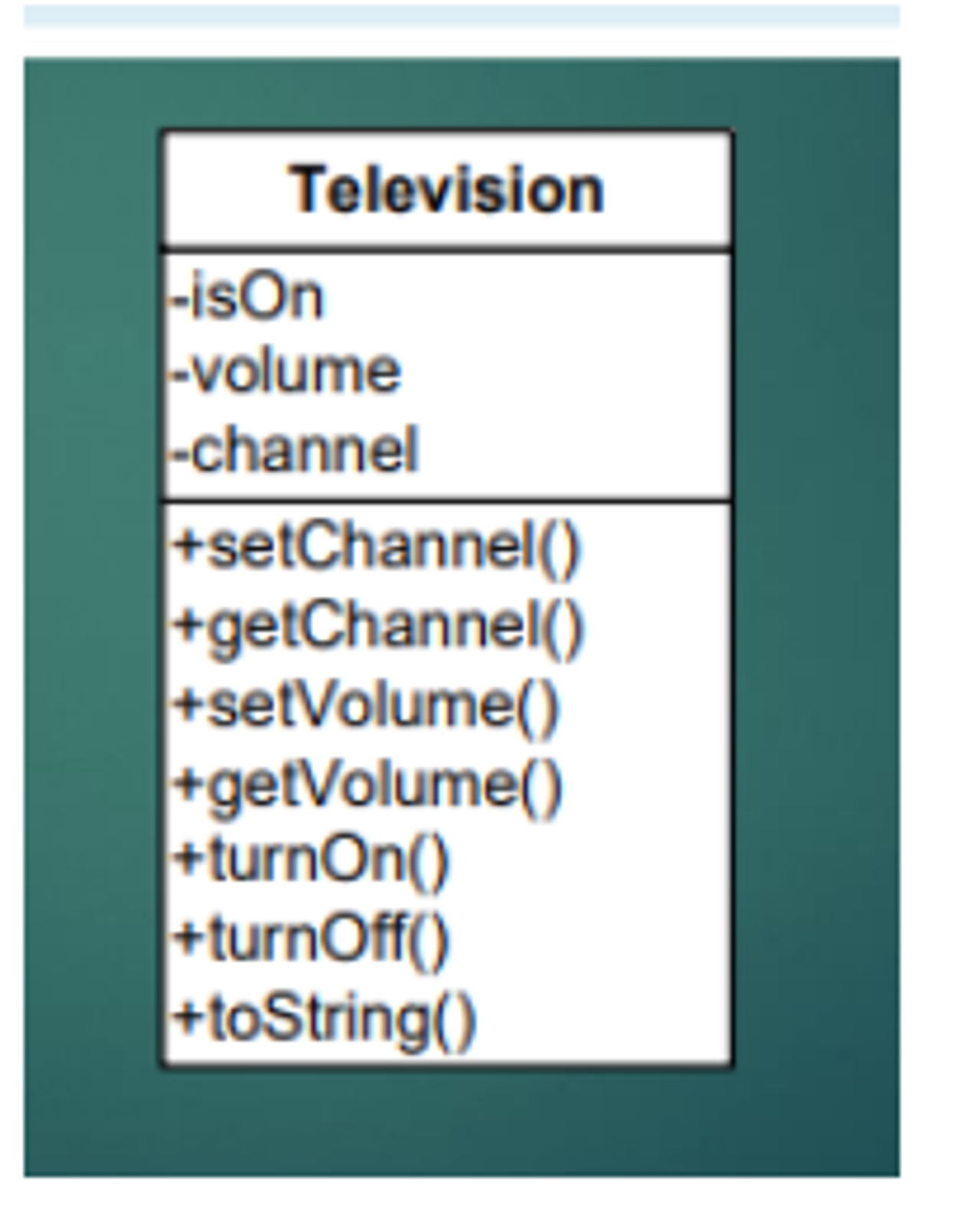
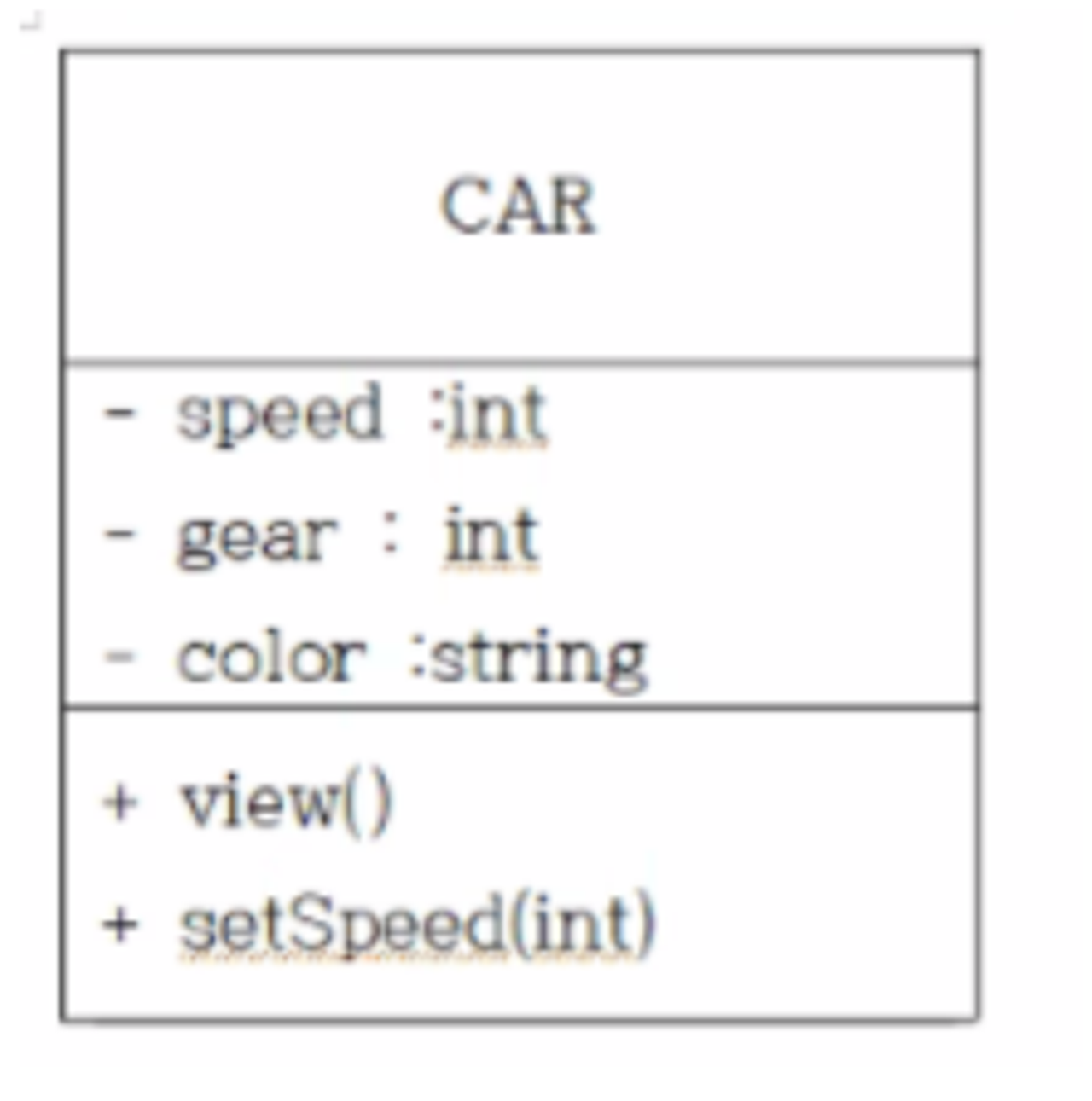
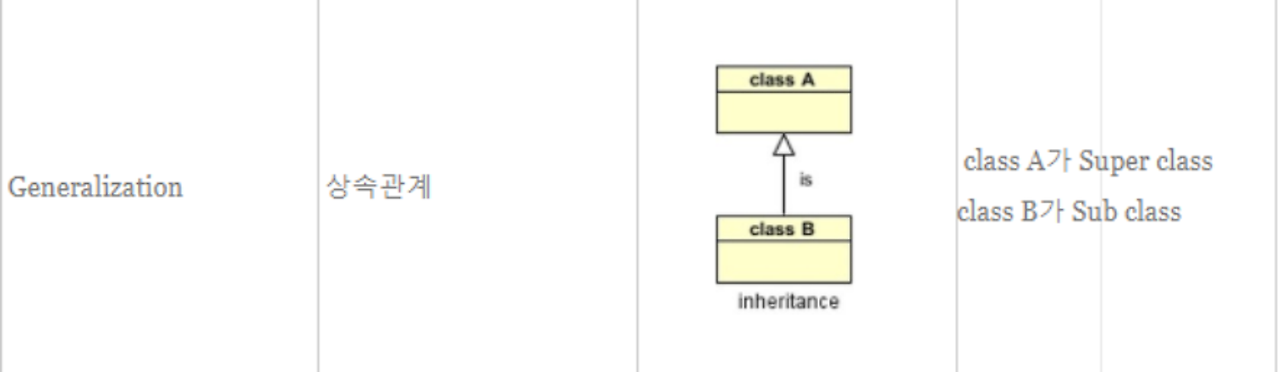
9. virtual κ°μν¨μ
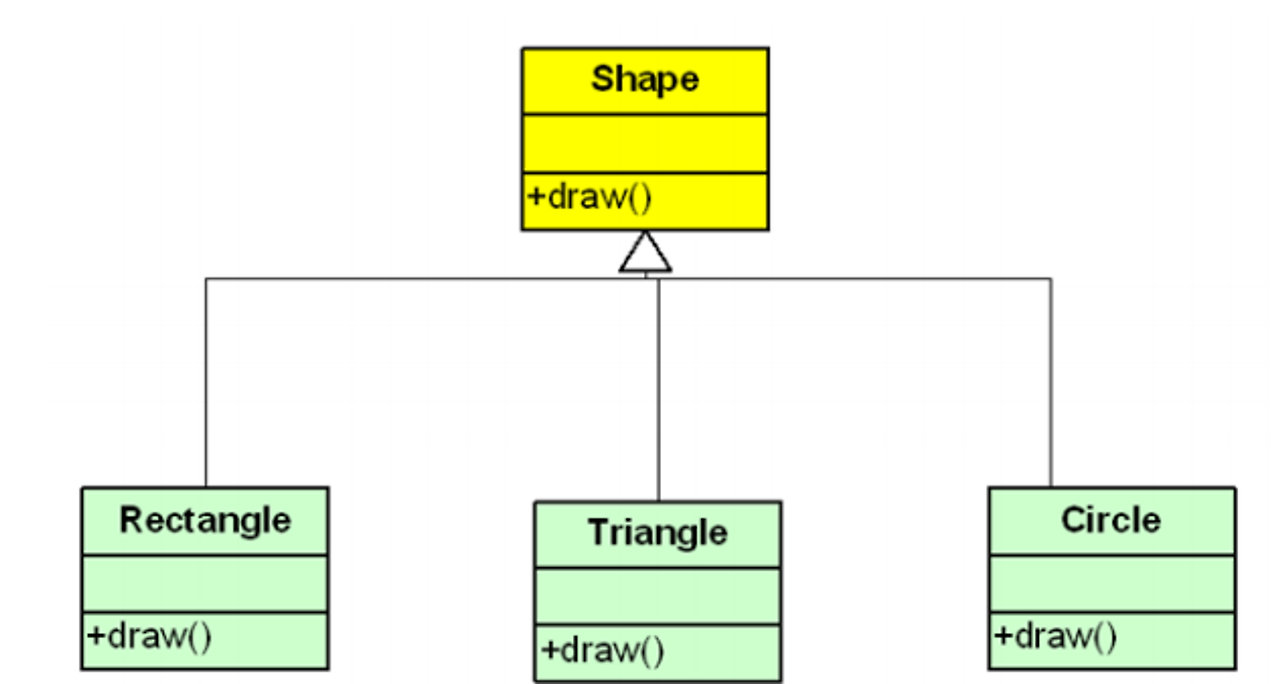
#include <iostream>
#include <string>
using namespace std;
class Shape {
protected:
int x, y;
public:
void setOrigin(int x, int y){
this->x = x;
this->y = y;
}
virtual void draw() { //κ°μ ν¨μ μ μ
cout <<"Shape Draw" << endl;
}
};
class Rectangle : public Shape { //μ¬μ μ
void draw() {
cout << "Rectangle Draw" << endl;
}
int main()
{
Shape *ps = new Rectangle();
ps->draw(); // rectangle draw()
delete ps;
Shape *ps1 = new Circle();
ps1->draw();
delete ps1;
return 0;
//λͺ¨λ λνμ λΆλͺ¨ν΄λμ€ ν¬μΈν°λ‘ κ°λ¦¬ν€κ³ μ΄ ν¬μΈν°λ‘
//draw() ν¨μλ₯Ό νΈμΆνλ©΄ μ¬μ μλ ν¨μκ° νΈμΆλ¨ (virtaul)
}
#include<iostream>
#include<list>
using namespace std;
class Shape {
protected:
int x, y;
public:
void setOrigin(int x, int y) {
this->x = x;
this->y = y;
}
virtual void draw() { // κ°μν¨μ
cout << "Shape Draw" << endl;
}
};
class Rectangle : public Shape {
protected:
int width, height;
public:
void setWidth(int w) {
this->width = w;
}
void setHegith(int h) {
this->height = h;
}
void draw() {
cout << "Rectangle Draw" << endl;
}
};
class Circle : public Shape {
protected:
int radius;
public:
void setRadius(int r) {
this->radius = r;
}
void draw() {
cout << "Circle Draw" << endl;
}
};
void main() {
Shape* pc = new Shape();//κ°μ²΄μμ±
pc->draw();
//Shape draw()
Shape* pb = new Rectangle();
pb->draw(); //κ°μν¨μλ‘ rectangle μ draw ()
Shape* pa = new Circle();
pa->draw(); //κ°μν¨μλ‘ circle μ draw ()
Rectangle* pr = (Rectangle*)pb; //νν₯νλ³ν1
pr->draw();
// rectangle draw()
((Rectangle*)pb)->draw();//νν₯νλ³ν1
// rectangle draw()
/*
Rectangle r1;
Shape &s1 = r1;
s1.draw();
*/
return;
}
λ€νμ±μ μ¬μ©νλ κ³Όμ μμ μλ©Έμλ₯Ό virtualλ‘ ν΄μ£Όμ§ μμΌλ©΄ λ¬Έμ κ° λ°μνλ€.
ο· (μμ ) String ν΄λμ€λ₯Ό μμλ°μμ κ° μ€μ μκ³Ό λ€μ ν€λλ₯Ό λΆμ΄λ MyString μ΄λΌλ ν΄λμ€λ₯Ό μ μνμ¬ λ³΄μ.
ο· String ν΄λμ€λ λ΄λΆμ λ¬Έμμ΄μ μ μ₯νκΈ° μν΄ char λ°°μ΄μ λμ μΌλ‘ μμ±νλ€.
ο· μλ©Έμμμλ μ΄ λμ μΌλ‘ μμ±λ 곡κ°μ λ°λ©νλ€.
10. λ°μΈλ© =κ°μν¨μκ° μμΌλ©΄ λμ λ°μΈλ©μ

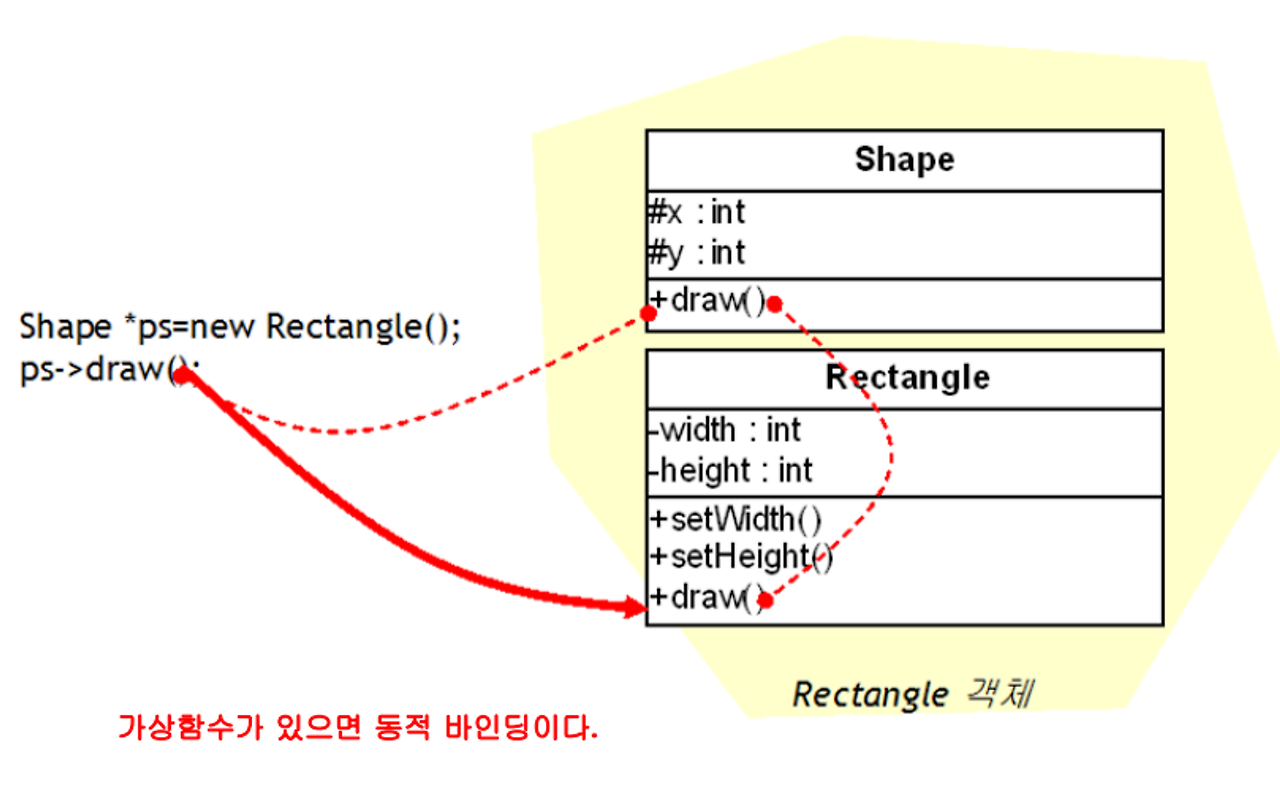
class Triangle: public Shape {
private:
int base, height;
public:
void draw() {
cout << "Triangle Draw" << endl;
}
};
int main() {
Shape *arrayOfShapes[3];
arrayOfShapes[0] = new Rectangle();
arrayOfShapes[1] = new Triangle();
arrayOfShapes[2] = new Circle();
for (int i = 0; i < 3; i++) {
arrayOfShapes[i]->draw();
}
}
#include <iostream>
using namespace std;
class Animal
{
public:
virtual void speak() { cout <<"Animal speak()" << endl; }
};
class Dog : public Animal
{
public:
void speak() { cout <<"λ©λ©" << endl; }
};
class Cat : public Animal
{
public:
void speak() { cout <<"μΌμΉ" << endl; }
};
int main()
{
Dog d;
Animal &a1 = d;
a1.speak();
Cat c;
Animal &a2 = c;
a2.speak();
return 0;
}
11. κ°μμλ©Έμ
//λ€νμ± μ¬μ©μ μλ©Έμ virtual λ‘ μνλ©΄
//Parent() μλ©Έμ λμ
//Child() μλ©Έμ λμ μν¨
#include <iostream>
using namespace std;
class Parent
{
public:
//~Parent() { cout << "Parent μλ©Έμ" << endl; }
virtual ~Parent() { cout << "Parent μλ©Έμ" << endl;}
};
class Child : public Parent
{
public:
~Child() { cout << "Child μλ©Έμ" << endl; }
};
int main()
{
Parent* p = new Child(); // μν₯ νλ³ν
delete p;
}
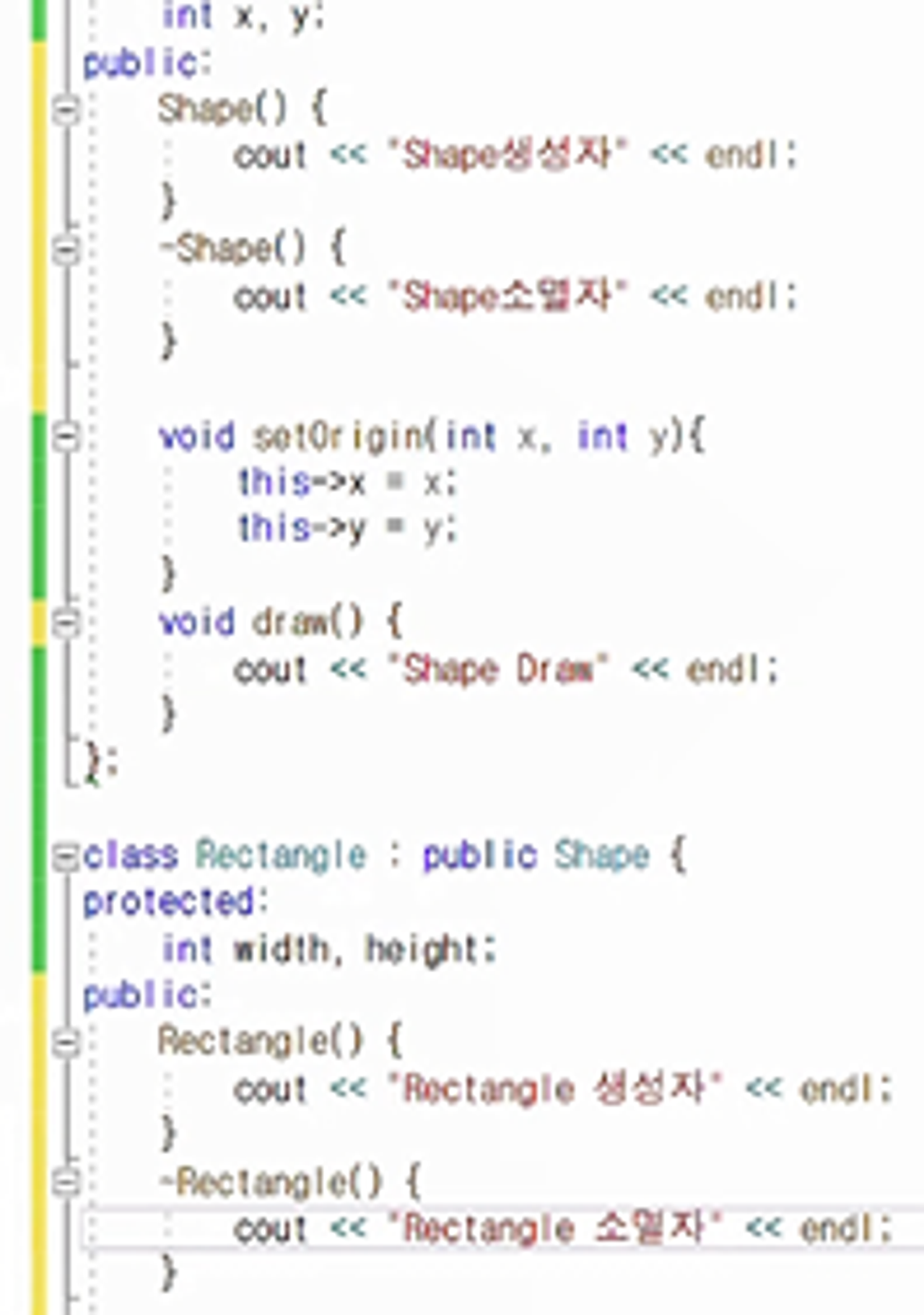
12. μμ κ°μν¨μ ⇒ μΆμν΄λμ€!!
virtual λ°νν ν¨μμ΄λ¦ (λ§€κ°λ³μ 리μ€νΈ) = 0;
virtual voud draw() = 0;
class Shape {
protected:
int x, y;
public:
…
virtual void draw() = 0; //μμ κ°μν¨μ
};
class Rectangle : public Shape {
private:
int width, height;
public:
void draw() {
cout << "Rectangle Draw" << endl;
}
};
int main()
{
Shape *ps = new Rectangle(); // OK!
ps->draw(); // Rectangleμdraw()κ°νΈμΆλλ€.
delete ps;
return 0;
}

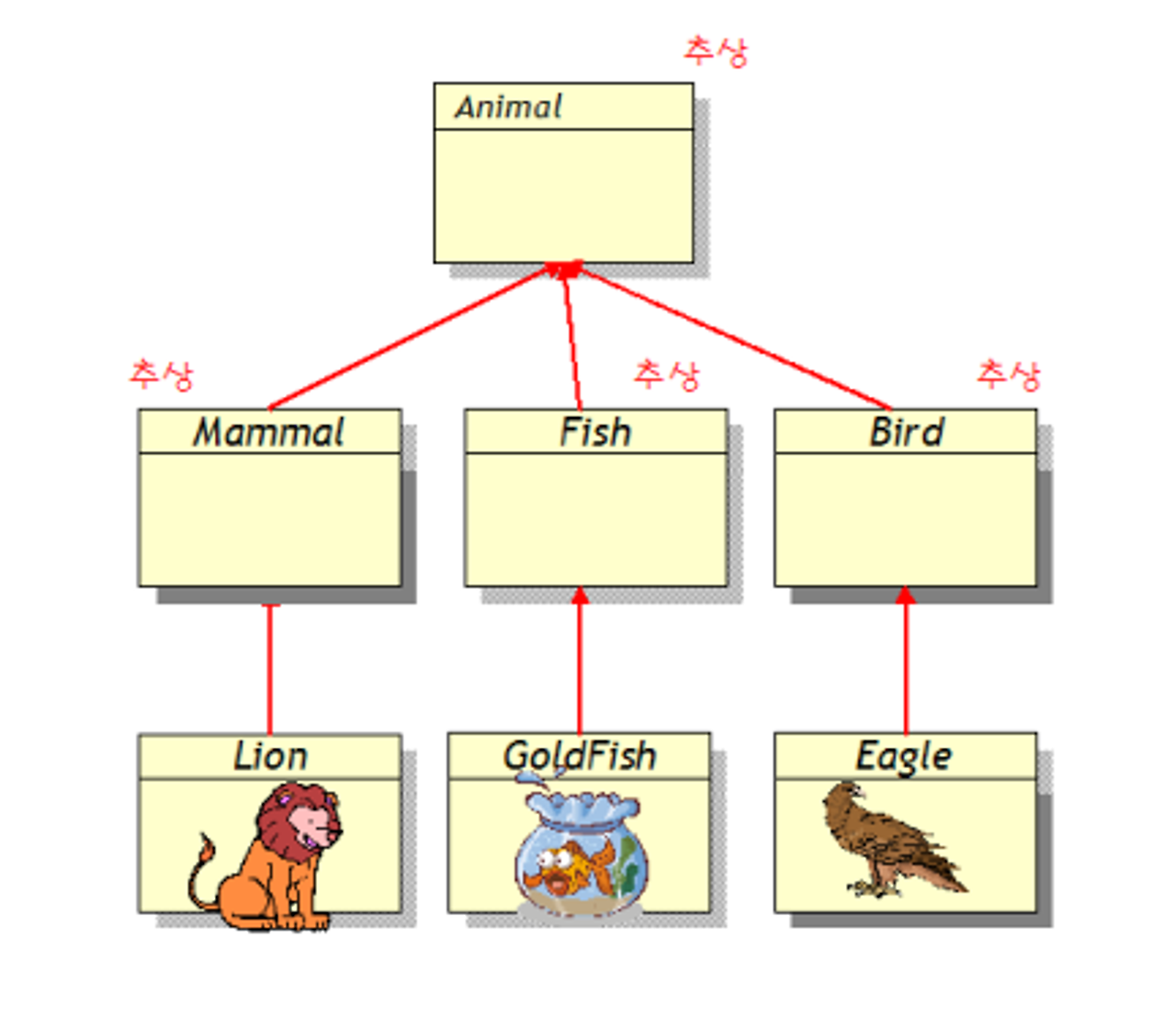
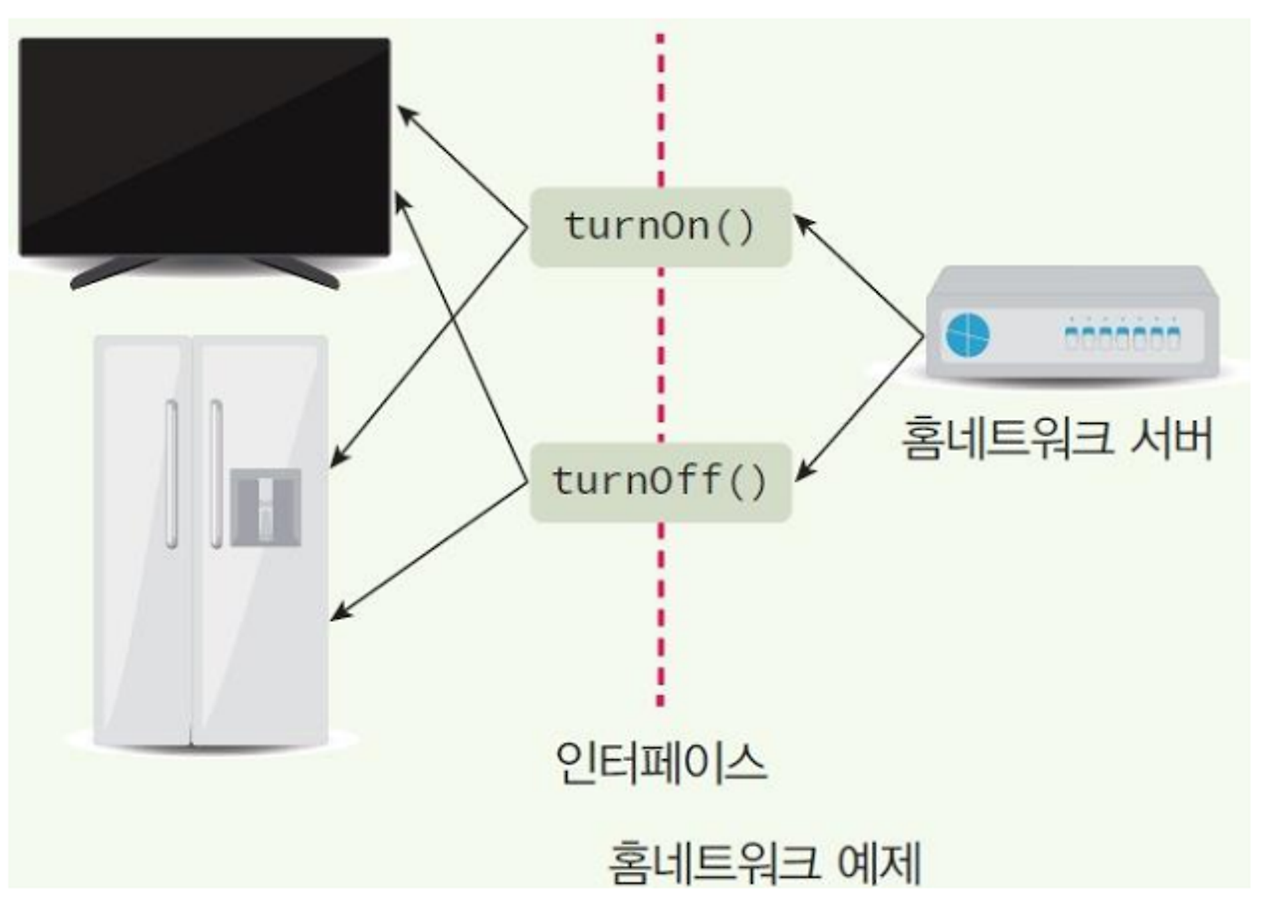
class Animal {
virtual void move() = 0;
virtual void eat() = 0;
virtual void speak() = 0;
};
class Lion : public Animal {
void move(){
cout << "μ¬μμ move() << endl;
}
void eat(){
cout << "μ¬μμ eat() << endl;
}
void speak(){
cout << "μ¬μμ speak() << endl;
}
};
class RemoteControl {
// μμκ°μν¨μμ μ
virtual void turnON() = 0; // κ°μ μ νμμΌ λ€.
virtual void turnOFF() = 0; // κ°μ μ νμλλ€.
}
class Television : public RemoteControl {
void turnON()
{
// μ€μ λ‘TVμμ μμμΌκΈ°μνμ½λκ°λ€μ΄κ°λ€.
...
}
void turnOFF()
{
// μ€μ λ‘TVμμ μμλκΈ°μνμ½λκ°λ€μ΄κ°λ€.
...
}
}
int main()
{
RemoteControl* r1 = new Television();
r1->turnOn();
RemoteControl* r2 = new Radio();
r2->turnOn();
((Television*)r1)->volCh();
((Radio*)r2)->volAMFM();
delete r1;
delete r2;
return 0;
}
13. μ€νΈλ¦Ό, μ μΆλ ₯(νμΌ μ½κ³ μ°κΈ°)

//μ
λ ₯
ifstream is;
is.open("score.txt");
int number;
is >> number;
//μΆλ ₯
ofstream os;
os.open("result.txt");
os << number
//score.txt
20100001 νκΈΈλ 100
20100002 κΉμ μ 90
20100003 κ°κ°μ°¬ 80
//Result.txt
20100001 νκΈΈλ 100
c > μ¬μ©μ > μμ μ»΄ν¨ν°μ΄λ¦ > source > repos > project
#include<iostream>
#include<fstream>
using namespace std;
int main() {
int number;
char name[30];
int score;
ifstream is;
is.open("input.txt");
if (!is) {
cerr << " νμΌ μ€νμ μ€ν¨ " << endl;
exit(1);
// λ¬Έμ μκΈ°λ©΄ μλ €μ€
}
is >> number >> name >> score; //isμ κ°λ€μ΄ λ€μ΄κ°(νλ) νμΌμ½μ
ofstream os; //
os.open("result.txt");
os << number << " " << name << " " << score << endl; // μ°λλΆλΆμ λμ΄μ°κΈ°ν΄μ£Όλ©΄λ¨
is >> number >> name >> score;
os << number << " " << name << " " << score << endl;
is.close();
os.close();
return 0;
}
//μ€νμν€λ©΄ result νμΌ μκΈ°λ©΄μ νλκ° λ€μ΄κ°μμ
#include<iostream>
#include<fstream>
using namespace std;
int main() {
int number;
char name[30];
int score;
ifstream is;
is.open("input.txt");
if (!is) {
cerr << " νμΌ μ€νμ μ€ν¨ " << endl;
exit(1);
// λ¬Έμ μκΈ°λ©΄ μλ €μ€
}
ofstream os; //
os.open("result.txt");
for (int i = 0; i < 3; i++) { //μκΈ° κ° λ²μ λμ§ μκΈ°
is >> number >> name >> score; //isμ κ°λ€μ΄ λ€μ΄κ°(νλ) νμΌμ½μ
os << number << " " << name << " " << score << endl; // μ°λλΆλΆμ λμ΄μ°κΈ°ν΄μ£Όλ©΄λ¨
}
is.close();
os.close();
return 0;
}
//νμΌ μ°κΈ°
int main()
{
ofstream os{ "numbers.txt" };
if (!os) {
cerr << "νμΌ μ€νμ μ€ν¨νμμ΅λλ€" << endl;
exit(1);
}
for(int i=0;i<100; i++)
os << i <<" ";
return 0;
// κ°μ²΄ osκ° λ²μλ₯Ό λ²μ΄λλ©΄ ofstream μλ©Έμκ° νμΌμ λ«λλ€.
int main()
{
ofstream os{ "numbers.txt" };
if (!os) {
cerr << "νμΌ μ€νμ μ€ν¨νμμ΅λλ€" << endl;
exit(1);
}
for(int i=0;i<100; i++)
os << i <<" ";
return 0;
// κ°μ²΄ osκ° λ²μλ₯Ό λ²μ΄λλ©΄ ofstream μλ©Έμκ° νμΌμ λ«λλ€.
}
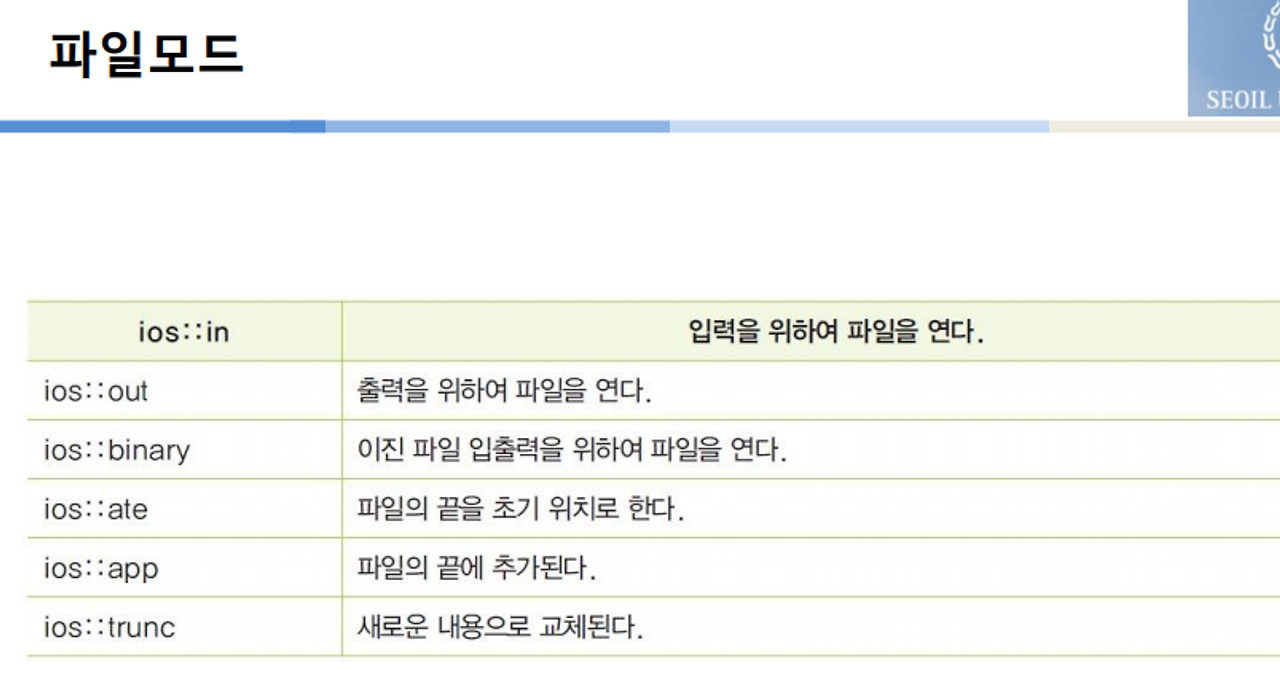
//νμΌλͺ¨λ μμ
int main()
{
using namespace std;
ofstream os(“input.txt", ios::app);
if (!os)
{
cerr << "νμΌ μ€νμ μ€ν¨νμμ΅λλ€" << endl;
exit(1);
}
os << "μΆκ°λλ μ€ #1" << endl;
os << "μΆκ°λλ μ€ #2" << endl;
return 0;
}
#include<iostream>
#include<fstream>
using namespace std;
int main() {
ofstream os{ "input.txt", ios::app }; //appλ λ€μ κ°μ μΆκ°
//μ΄λ‘μμ μμ½μ΄
if (!os) {
cerr << "νμΌμ€ν μ€ν¨" << endl;
exit(1);
}
os << "μΆκ° μ€ #1" << endl;
os << "μΆκ° μ€ #2" << endl;
os.close();
}
#include<iostream>
#include<fstream>
using namespace std;
int main() {
ifstream is { "input.txt"}; //appλ λ€μ κ°μ μΆκ°
//μ΄λ‘μμ μμ½μ΄
if (!is) {
cerr << "νμΌμ€ν μ€ν¨" << endl; //cerr μλ¬μ½λμ‘μλ
exit(1);
}
int hour;
double temp;
while (is >> hour >> temp) {
cout << hour << "μ : μ¨λ :" << temp << endl;
}
is.close();
}
//λ°μ΄ν° μ²λ¦¬ μμ
#include <iostream>
#include <fstream>
using namespace std;
int main()
{
ifstream is{ “input.txt" };
if (!is) { // ! μ°μ°μ μ€λ²λ‘λ©
cerr << "νμΌ μ€νμ μ€ν¨νμμ΅λλ€" << endl;
exit(1);
}
int hour;
double temperature;
while (is >> hour >> temperature) {
cout << hour << "μ: μ¨λ "<< temperature << endl;
}
return 0;
}
//λ²‘ν° μ΄μ© μμ
#include<vector>
class TempData {
public:
int hour;
double temperature;
};
int main()
{
ifstream is{ “input.txt" };
if (!is) { // ! μ°μ°μ μ€λ²λ‘λ©
cerr << "νμΌ μ€νμ μ€ν¨νμμ΅λλ€" << endl;
exit(1);
}
vector<TempData> temps;
int hour;
double temperature;
while (is >> hour >> temperature) {
temps.push_back(TempData{ hour, temperature });
}
for ( TempData t : temps) {
cout << t.hour << "μ: μ¨λ " << t.temperature << endl;
}
return 0;
}
#include<iostream>
#include<list>
#include<iostream>
#include<fstream>
using namespace std;
void main() {
ifstream is{ "input.txt" };
ofstream os{ "output.txt" , ios::app}; /
if (!is) {
cerr << "νμΌμ€νμ€ν¨" << endl;
exit(1);
}
int hour;
double temp;
while (is >> hour >> temp) {
os << hour << "μ : μ¨λ :" << temp << endl;
}
is.close();
os.close();
//μ½κ³ μ°κΈ° (input μμ μ½κ³ output μμ μ°κΈ°)
return;
}
#include<vector>
int main (){
class TempData{
public:
int hour;
double temp;
}
ifstream is { "input.txt"}; //appλ λ€μ κ°μ μΆκ°
//μ΄λ‘μμ μμ½μ΄
if (!is) {
cerr << "νμΌμ€ν μ€ν¨" << endl; //cerr μλ¬μ½λμ‘μλ
exit(1);
}
vector< TempData > temps; //ν΄λμ€ μ체λ₯Ό μ§μ΄λ£μ μ μμ
int hour;
double temp;
while (is >> hour >> temp) {
temps.push_back(TempData {hour,temp});
}
for(TempData t:temps) //λ°λ³΅μ
cout<<t.hour<<"μ:μ¨λ"<<t.temp<<endl;
is.close();
return 0;
}
벑ν°λ₯Ό λ§λ€μ΄μ κ°μ κ°μ Έμμ κ°μ μ§μ΄ λ£μ
벑ν°μ λ€ μ§μ΄λ£μ μκ° μ¨λ μκ° μ¨λ μκ° μ¨λ
ν΄λμ€λΌμ & λΉ μ§ (λ°λ³΅μ)λ₯Ό ν΅ν΄ κ°μ κ°μ Έμ΄
#include<vector>
int main (){
class TempData{
public:
int hour;
double temp;
}
ifstream is { "input.txt"}; //μμ€νμΌκ³Ό κ°μ΄ μλ κ²½λ‘μμ΄γ
γ
μΌν¨
//μ΄λ‘μμ μμ½μ΄
if (!is) {
cerr << "νμΌμ€ν μ€ν¨" << endl;
exit(1);
}
int num;
while (is){
is>>num;
cout<<num << " ";
}
cout << endl;
is.close();
return 0;
}
14. μ’ν(μ λκ²½λ‘/μλκ²½λ‘)
ν¨μμ νμΌλͺ λ§ μ§μ νλ©΄ νμ¬ μμ λλ ν°λ¦¬(working directory)μμ νμΌμ μ°λ€
fopen("hello.txt", "w") νμ¬ νμΌ μ€ν
- μ λ κ²½λ‘ : ifstream is("c:\\in\\input");
- μλ κ²½λ‘ : ifstream is{"in\\input.txt"};
μλ κ²½λ‘λ μμ
λλ ν°λ¦¬λ₯Ό κΈ°μ€μ νμΌ
- μλκ²½λ‘ κΈ°μ€ ..\\ λ λΆλͺ¨λλ ν 리λ₯Ό λ»ν¨
• ifstream is{ "..\\in\\input.txt" };
-
리λ
μ€ λ° OS Xλ κ²½λ‘λ₯Ό ννν λ \ λμ /λ₯Ό μ¬μ©
• fopen("/home/project/hello.txt", "w");
..\\
..\\..\\
15. νλλ
class MyClass
{
friend void sub();
//friend ν΄λμ€μ΄λ¦ ν¨μμ΄λ¦(λ§€κ°λ³μλͺ©λ‘);
...
};
#include <iostream>
#include <string>
class Company {
private:
int sales;
int profitl
// sub()λCompanyμ μ μ©λ©€λ²μ μ κ·Όν μ μλ€.
pubilc:
friend void sub(Company& c);
company() : sales(0), profit(100) {}
}; //μ»΄νΌλ μμ μΉκ΅¬ λ§λ¬
void sub(Company& c) { //cκ°μ²΄κ° μ κ·Όκ°λ₯νλ μ€νλ¨
cout<< c.profit << endl;
}
int main {
Company c1; //μ€νμν€λ©΄μ
sub(c1); // κ°μ²΄ μ§μΈνμλ
return 0;
}
μλμ λ©λͺ¨λ¦¬ : μ€ν λ©λͺ¨λ¦¬ / λΉ λ₯΄λ€
λλμ λ©λͺ¨λ¦¬ : ν λ©λͺ¨λ¦¬ / κ΄νν λ©λͺ¨λ¦¬ μ¬μ©κ°λ₯